A Blog providing enriching content to learn and become professional developer in Ruby on Rails.
Sunday 21 December 2014
Saich RoR Professional Blog: Ruby on Rails Interview Questions
Ruby on Rails Interview Questions
1. what is the use of filters in rails
2. whats is the use of partials in rails
3. usage of redirect and redirect_to in rails
4. whats is REST and RESTful routes in rails
5. What is use of associations in rails
6. how many types of associations are there in rails
7. what is the use of callbacks in rails
8. when we use polymorphic associations in rails
9. how can we define roles in rails application
10. how many types of constructors are there in ruby on rails
11. what is the purpose of using attr_accesser in rails
12. what is the purpose of using devise gem and cancan in rails application
13. How can we use before_filter callback in controller
14. what is the purpose using around filter in rails
15. how to find max difference and min difference between elements in Array
16. see below array
marks1 = [ ['Sai', 43], ['Benny', 9], ['Ajay',53] ]
marks2 = ['Praveen', 87], ['Nag', 5],['Vamsi',56],['Janu', 55] ]
who has got maximum marks in above array
who has got minimum marks in above array
17. What is difference between Rails2.X, Rails3.X and Rails4.x
18. which way we use helper methods in our rails application
19. difference between blank? and empty? methods in rails
20. What is the difference between Ruby’s
Hash
and ActiveSupport’sHashWithIndifferentAccess
?21. What is the purpose of using Strong Parameters in Rails
22. What are HTTP Verbs in Rails
23. What is CSRF? How does Rails protect against it?
24. What is Active Record and what is Arel? Describe the capabilities of each.
25. What is the Convention over Configuration pattern? Provide examples of
how it is applied in Rails.
Note: For any queries or to know answers drop a message to this " chsai.btech@gmail.com " email...!
Please forward the content to my Email ID anything find new in Rails.
Saturday 20 December 2014
REST and RESTful Routes in Rails
What is REST?
- Representational State Transfer
- Don't perform procedures
- Perform sate transformations upon resources
Why do we need use REST?
- Default routes (using hashes) works
- REST is Rails default; optimized for it
- Most professional Rails developers use REST
- Gives an application an API for free(HTML,XML,JSON etc.,)
- Provides conventions,consistent structure, and simplicity
- Improves application security
REST Paradigm Requirements
- Organize code into resources - Rails encourages one controller for each model
- Learn HTTP verbs for working with resources - Consider which CRUD actions are suited for each
- Map a new URL syntax to controller actions - Change Rails routes
- Modify existing links and forms to use new URL syntax - Learn about RESTful route helpers
REST in Rails
<form method="PATCH" action="/subjects/1243">
#......
</form>
<form method="POST" action="/subjects/1243">
<input type="hidden" name="_method" value="patch"/>
#......
</form>
RESTful Routes:
# config/routes.rb
resources :subjects do
member do
get :delete
end
end
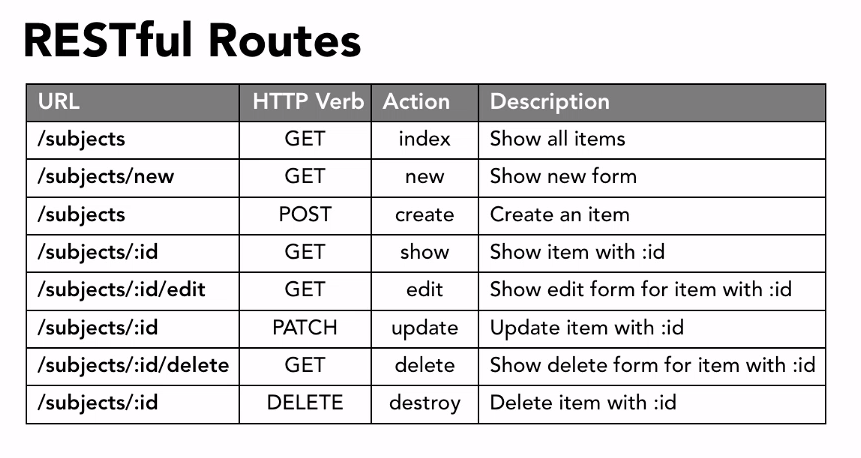
To know routes in your application: rake routes
RESTful Links and Forms
RESTful URL Helpers
{:controller => 'subjects', :action => 'show', :id =>5}
subject_path(5)
<%= link_to ('All Subjects', subjects_path) %>
<%= link_to ('Show Subject', subject_path(@subject.id)) %>
<%= link_to ('Edit Subject', edit_subject_path(@subject.id)) %>
for additional arguments use hash
<%= link_to ('Edit Subject',
edit_subject_path(@subject.id, :page => 1, :sort => 'name')) %>
REST Form Helpers
form_for(:subject, :url => subject_path(@subject.id),
:html => {:method => :patch}) do
#...........
end
form_for(@subject) do |f|
#.....
end
Using Non-Standard Resources
resources :admin_users, :except => [:show]
resources :products, :only => [:index, :show]
Using Non-Standard Actions
resources :subjects do
member do
get :delete # delete_subject_path(:id)
end
collection do
get :export # export_subjects_path
end
end
Nested Resources
resources :subjects do
member do
get :delete
end
resources :pages do
member do
get :delete
end
end
end
REST URL Helpers
<%= link_to('All Pages', subject_pages_path(@subject.id)) %>
<%= link_to('Show Page', subject_page_path(@subject.id, @page.id)) %>
<%= link_to('Edit Page',
edit_subject_page_path(@subject.id, @page.id)) %>
<%= link_to('All Sections',
subject_page_sections_path(@subject.id, @page.id)) %>
<%= link_to('Show Section',
subject_page_section_path(@subject.id, @page.id,@section.id)) %>
My First Personal Ruby Programm....!
sa = "\u0c38"
aa = "\u0c3E"
puts sa + aa
ya = "\u0c2F"
ii = "\u0c3F"
puts ya + ii
saa = sa + aa
i = ya + ii
puts saa + ' ' + i
# file open : vim sai.rb
#compile : ruby sai.rb
# output :
సా
యి
సా యి
![]() |
Saich - RubyonRails Developer |
Find me in Web
Github
Bitbucket
Blogsopt
Rails Asset Pipeline - How Handle Assets
Rails 3.1 introduced the Asset Pipeline, a way to organize and process front-end assets. It provides an import/require mechanism (to load dependent files) that provides many features. While the Asset Pipeline does have its rough edges, it does solve and provide many of the modern best practices in serving these files under HTTP 1.1. Most significantly, the Asset Pipeline will:
- Collect, concatenate, and minify all assets of each type into one big file
- Version files using fingerprinting to bust old versions of the file in browser caches
The Asset Pipeline automatically brings with it Rails’ selection of Coffeescript as its JavaScript pre-processed / transpiled language of choice and SASS as its CSS transpiled language. However, being an extensible framework, it does allow for additional transpiled languages or additional file sources. For example, Rails Assets brings the power of Bower to your Rails apps, allowing you to manage third-party JavaScript and CSS assets very easily.
Friday 19 December 2014
Processing flow of a Rails Request
At the highest level, Rails requests are served through an application server, which is responsible for directing an incoming request into a Ruby process. Popular application servers that use the Rack web request interface include Phusion Passenger, Mongrel, Thin, and Unicorn.
Rack parses all request parameters (as well as posted data, CGI parameters, and other potentially useful bits of information) and transforms them into a big Hash (Ruby’s record / dictionary type). This is sometimes called the env hash, as it contains data about the environment of the web request.
In addition to this request parsing, Rack is configurable, allowing for certain requests to be directed to specific Rack apps. If you want, for example, to redirect requests for anything in your admin section to another Rails app, you can do so at the Rack level. You can also declare middleware here, in addition to being able to declare it in Rails.
Those requests that are not directed elsewhere (by you in Rack) are directed to your Rails app where it begins interacting with the Rails ActionDispatcher, which examines the route. Rails apps can be spit into separate Rails Engines, and the router sends the request off to the right engine. (You can also redirect requests to other Rack compatible web frameworks here.)
Once in your app, Rails middleware – or your custom middleware – is executed. The router determines what Rails controller / action method should be called to process the request, instantiates the proper controller object, executes all the filters involved, and finally calls the appropriate the action method.
Further detail is available in the Rails documentation.